http服务器基础代码
1 2 3 4 5 6
| var http = require('http')
http.createServer(function (req, res) {
res.end(); }).listen(8000, '127.0.0.1')
|
注意其中res.end()
一定不能缺少,否则服务器会以为请求没有结束,一直处于等待状态。
启动服务器

解析表单Get请求
引入url
模块
1 2 3 4 5 6 7 8
| var http = require('http') var url = require('url')
http.createServer(function (req, res) { var uu = url.parse(req.url) console.log(uu) res.end(); }).listen(8000, '127.0.0.1')
|
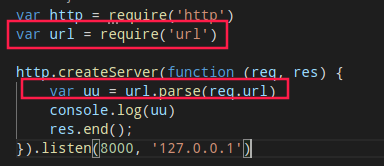
发起请求
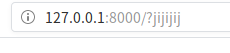
打印结果:
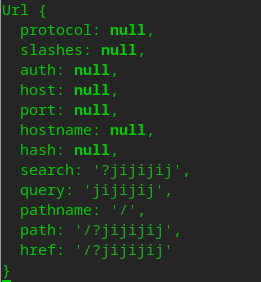
所以用
1
| url.parse(req.url).query
|

可以解析到

引入querystring
模块
1 2 3
| var querystring = require('querystring') ...... var uu = querystring.parse(xxxxx)
|
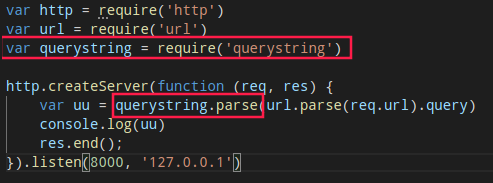
解析结果

解析表单POST请求
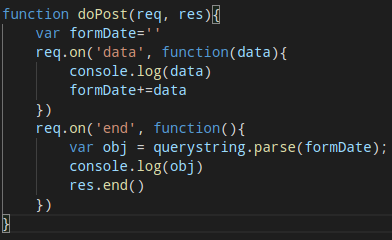
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| function doPost(req, res){ var formDate=''
req.on('data', function(data){ console.log(data) formDate+=data }) req.on('end', function(){ var obj = querystring.parse(formDate); console.log(obj) res.end() }) }
|
由于post请求发送的数据可能很大,所以接收过程分为两步,第一个req.on用于接收数据,第二个req.on用于判断post请求结束并做出相应处理,比如用res.end()结束请求链接。
分文件
在需要被引用的文件中写
在引用文件中写
var post=require(‘./doPost’)
socket链接
socket客户端
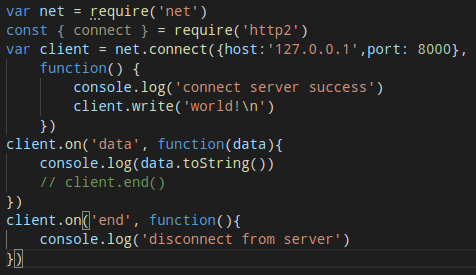
socket服务端
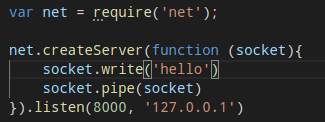
实时通讯(多人聊天室)
服务端:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| var net = require('net'); var clientList = [] net.createServer(function (socket){ clientList.push(socket) socket.write('hello') socket.on('data', function(data){ console.log(data.toString()) // socket.write(data) broadcast(data) }) socket.on('end', function(){ socket.write("bye") }) }).listen(8000, '127.0.0.1')
function broadcast(data){ for (var i = 0; i < clientList.length; i++){ clientList[i].write(data) } }
|
客户端
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| var net = require('net') var hostname = process.argv[2] var port = process.argv[3]
var client = net.connect({host: hostname, port: port}, function() { console.log('connect server success')
process.stdin.setEncoding('utf-8') process.stdin.resume() process.stdin.on('data', function(data) { if (data != null) { client.write('data:' + data) } }) }) client.on('data', function(data){ console.log(data.toString()) // client.end() }) client.on('end', function(){ console.log('disconnect from server') })
|
静态服务器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| var http = require('http') var fs = require('fs') var path = require('path') var mime = require('mime') const { type } = require('os') var cache = {}
function send404(response) { response.writeHead(404, {'Content-Type': 'text/plain'}) response.write('Error 404: resource not found') response.end() }
function sendFile(response, filePath, fileContents) { var ss = mime.getType(path.basename(filePath)) + ';' + 'charset=utf-8' console.log(ss) response.writeHead( 200, {'Content-Type': ss} ); response.end(fileContents) }
function serverStatic(response, cache, absPath) { if(cache[absPath]) { sendFile(response, absPath, cache[absPath]) }else{ fs.exists(absPath, function(exists) { if (exists) { fs.readFile(absPath, function(err, data) { if(err){ send404(response) } else { cache[absPath] = data sendFile(response, absPath, data) } }) } else{ send404(response) } }) } }
var server = http.createServer(function(req, res) { var filePath = false;
if(req.url == '/') { filePath = 'public/index.html' } else { filePath = 'public' + req.url }
var absPath = './' + filePath
serverStatic(res, cache, absPath) })
server.listen(8000, '127.0.0.1')
|